TestNG Listeners in Selenium WebDriver can be implemented at two levels:
- Class level: In this, you implement listeners for each particular class, no matter how many test cases it includes.
- Suite level: In this, you implement listeners for a particular suite which includes several classes of test cases.
1. ITestListener
ITestListener is the most adopted TestNG listener in Selenium WebDriver. It provides you with an easy to implement interface through a normal Java class, where the class overrides every method declared inside the ITestListener. By using this TestNG listener in Selenium WebDriver, you can change the default behaviour of your test by adding different events to the methods. It also defines a new way of logging or reporting.
The following are some methods provided by this interface:
- onStart: This method is invoked before any test method gets executed. This can be used to get the directory from where the tests are running.
- onFinish: This method is invoked after all tests methods gets executed. This can be used to store information of all the tests that were run.
- onTestStart: This method is invoked before any test methods are invoked. This can be used to indicate that the particular test method has been started.
- onTestSkipped: This method is invoked when each test method is skipped. This can be used to indicate that the particular test method has been skipped.
- onTestSuccess: This method is invoked when any test method succeeds. This can be used to indicate that the particular test method has successfully finished its execution.
- onTestFailure: This method is invoked when any test method fails. This can be used to indicate that the particular test method has failed. You can create an event for taking a screenshot which will show where the test has been failed.
- onTestFailedButWithinSuccessPercentage: This method is invoked each time the test method fails but is within the success percentage mentioned. To implement this method, we use two attributes as a parameter of test annotation in TestNG, i.e.
successPercentage
andinvocationCount
. ThesuccessPercentage
takes the value of percentage of successful tests andinvocationCount
denotes the number of times that a particular test method executes. For example:@Test(successPercentage=60, invocationCount=5)
. In this annotation, the success percentage is 60% and the invocation count is 5, which means that out of 5 times, if the test method gets passed at least 3 times ((⅗)*100= 60), it will be considered as passed.
For every ITestListener method we usually pass the following arguments:
- “ITestResult” interface along with its instance, “result,” which describes the result of a test. Note: If you want to trace your exception through ITestResult then you need to avoid try/catch handling.
- “ITestContext” interface along with its instance “context” which describes the test context containing all the information of the given test run.
package TestNgListeners;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class ListenersBlog implements ITestListener {
public void onTestStart(ITestResult result) {
System.out.println("New Test Started" + result.getName());
}
public void onTestSuccess(ITestResult result) {
System.out.println("Test Successfully Finished" + result.getName());
}
public void onTestFailure(ITestResult result) {
System.out.println("Test Failed" + result.getName());
}
public void onTestSkipped(ITestResult result) {
System.out.println("Test Skipped" + result.getName());
}
public void onTestFailedButWithinSuccessPercentage(ITestResult result) {
System.out.println("Test Failed but within success percentage" + result.getName());
}
public void onStart(ITestContext context) {
System.out.println("This is onStart method" + context.getOutputDirectory());
}
public void onFinish(ITestContext context) {
System.out.println("This is onFinish method" + context.getPassedTests());
System.out.println("This is onFinish method" + context.getFailedTests());
}
}
Below is the code that includes the tests methods (TestNGListenersTest.java). Make sure you add a Listeners annotation just above your class name to implement the above added methods. Syntax:
package TestNgListeners;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class ListenersBlog implements ITestListener {
public void onTestStart(ITestResult result) {
System.out.println("New Test Started" + result.getName());
}
public void onTestSuccess(ITestResult result) {
System.out.println("Test Successfully Finished" + result.getName());
}
public void onTestFailure(ITestResult result) {
System.out.println("Test Failed" + result.getName());
}
public void onTestSkipped(ITestResult result) {
System.out.println("Test Skipped" + result.getName());
}
public void onTestFailedButWithinSuccessPercentage(ITestResult result) {
System.out.println("Test Failed but within success percentage" + result.getName());
}
public void onStart(ITestContext context) {
System.out.println("This is onStart method" + context.getOutputDirectory());
}
public void onFinish(ITestContext context) {
System.out.println("This is onFinish method" + context.getPassedTests());
System.out.println("This is onFinish method" + context.getFailedTests());
}
}
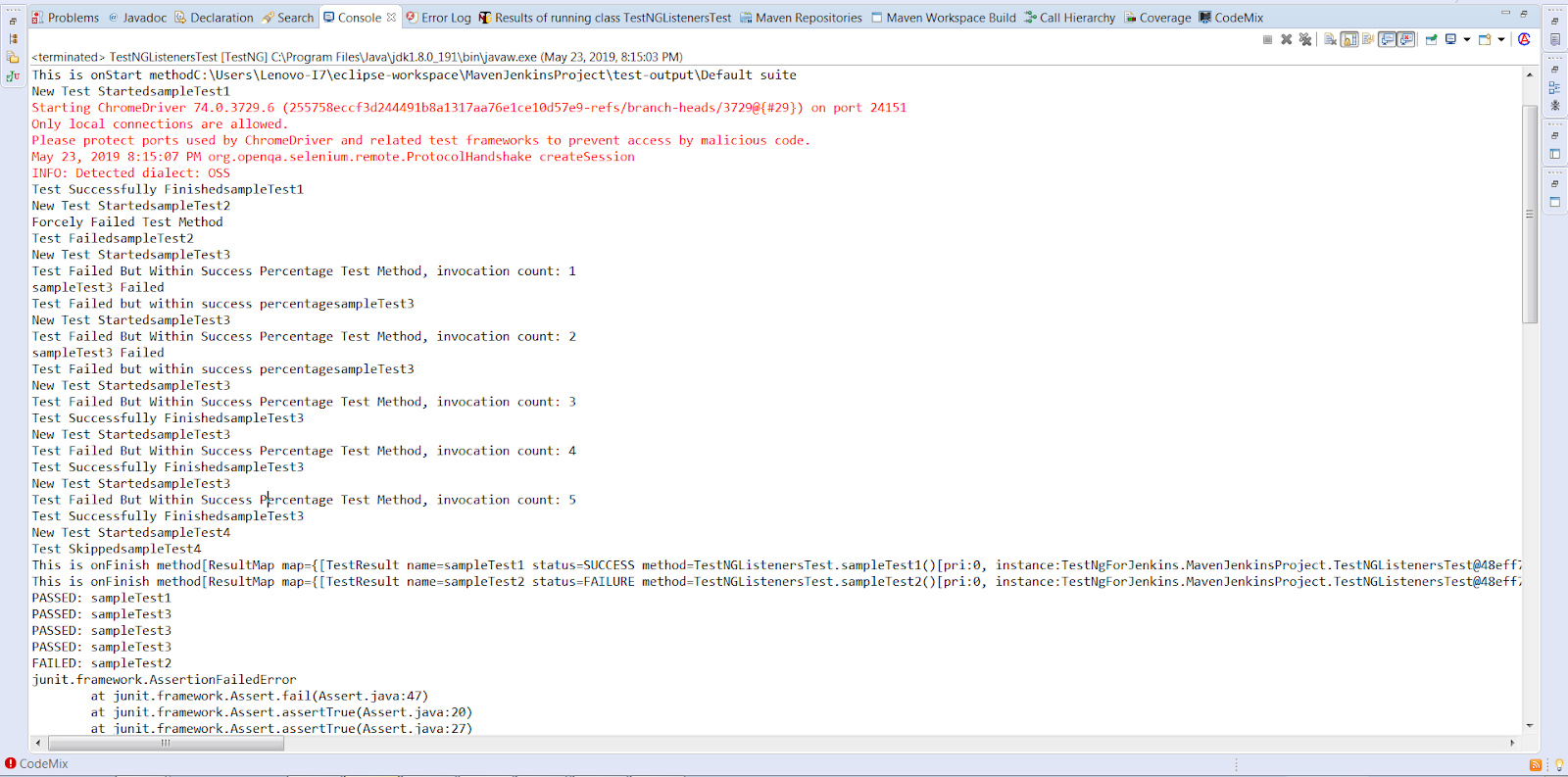
Now, suppose you have multiple classes in your project, then adding TestNG Listeners in Selenium WebDriver to each class might be a pain. In such cases, you can create a test suite and add a Listeners tag to your suite (XML file) instead of adding Listeners to each class. Here is the example code (testng.xml) for running the test at the suite level :
<suite name="TestNG Listeners Suite" parallel="false">
<listeners>
<listener class-name="TestNgListeners.ListenersBlog" /> </listeners>
<test name="Test">
<classes>
<class name="TestNgListeners.TestNGListenersTest" /> </classes>
</test>
</suite>
2. IInvokedMethodListener
This interface allows you to perform some action before and after a method has been executed. This listener gets invoked for configuration and test methods. This TestNG listener in Selenium WebDriver works the same as the ITestListerner and the ISuiteListerner. However, there is a difference that you should make a note of and that is that, in IInvokedMethodListener, it makes the call before and after every method.
There are two methods to be implemented:
beforeInvocation(): This method is invoked prior every method.
afterInvocation(): This method is invoked post every method.
InvokedMethodListeners.java(includes listeners implemented methods) package TestNgListeners;
import org.testng.IInvokedMethod;
import org.testng.IInvokedMethodListener;
import org.testng.ITestResult;
public class InvokedMethodListeners implements IInvokedMethodListener {
public void beforeInvocation(IInvokedMethod method, ITestResult testResult) {
System.out.println("Before Invocation of: " + method.getTestMethod().getMethodName() + "of Class:" + testResult.getTestClass());
}
public void afterInvocation(IInvokedMethod method, ITestResult testResult) {
System.out.println("After Invocation of: " + method.getTestMethod().getMethodName() + "of Class:" + testResult.getTestClass());
}
}
package TestNgListeners;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Listeners;
import org.testng.annotations.Test;
value = InvokedMethodListeners.class) public class InvokedMethodListenersTest { (
public void test1() {
System.out.println("My first test");
}
public void test2() {
System.out.println("My second test");
}
public void setUp() {
System.out.println("Before Class method");
}
public void cleanUp() {
System.out.println("After Class method");
}
}
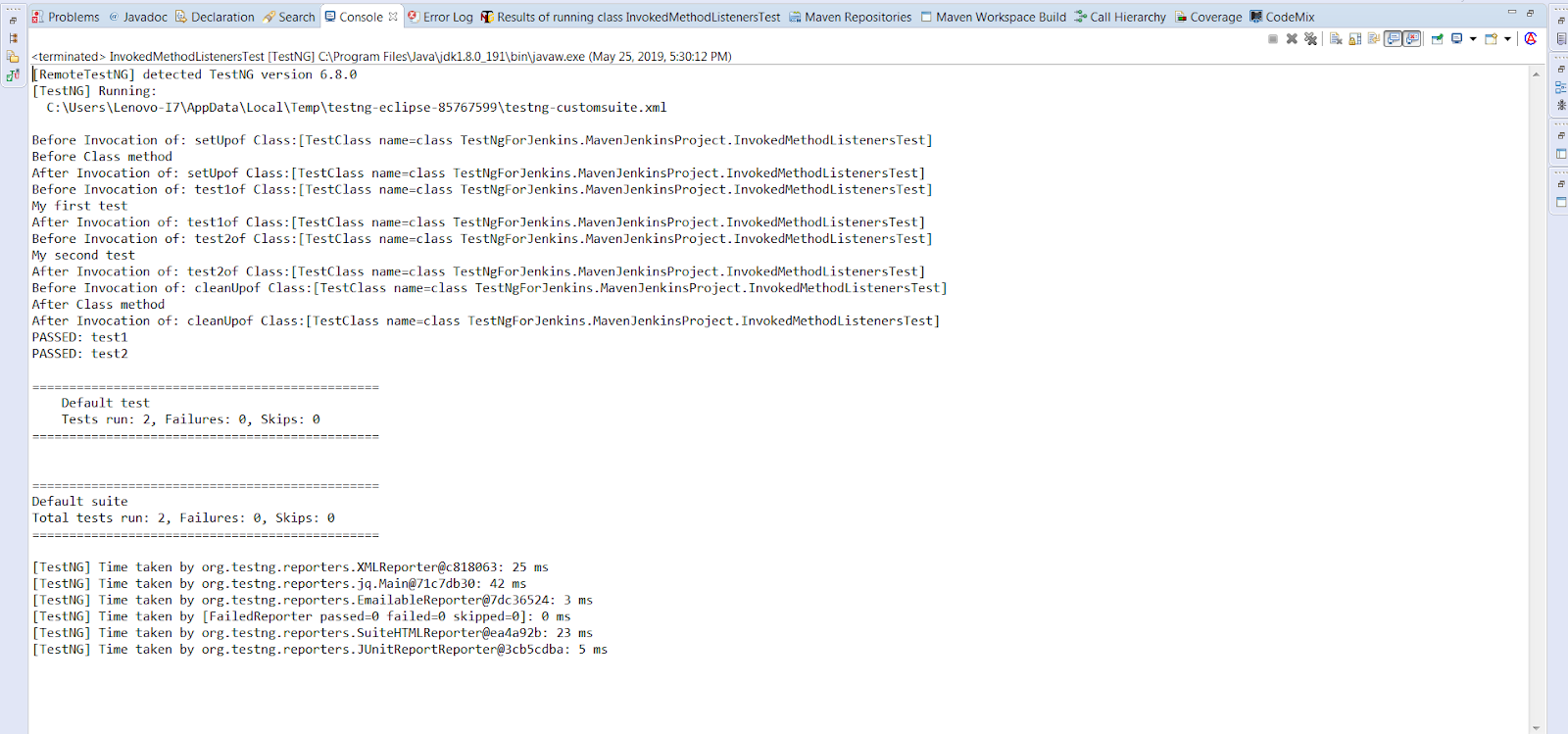
4. ISuiteListener
This TestNG listener in Selenium WebDriver is implemented at a suite level called ISuiteListener. It has two methods:
- onStart: This method is invoked prior the test suite execution.
- onFinish: This method is invoked post the test suite execution.
This listener basically listens to the events that have occurred before and after the execution of the suite. If the parent suite further contains child suites then child suites are executed before running the parent suite.
Step 1: Implementing ISuiteListener with normal java class and adding the unimplemented methods.
Class: SuiteListeners
package TestNgListeners;
import org.testng.ISuite;
import org.testng.ISuiteListener;
public class SuiteListeners implements ISuiteListener {
public void onStart(ISuite suite) {
System.out.println("Suite executed onStart" + suite.getName());
}
public void onFinish(ISuite suite) {
System.out.println("Suite executed onFinish" + suite.getName());
}
}
5. IReporter
This TestNG listener in Selenium WebDriver provides an interface which helps you to customize the test report generated by TestNG. It provides the
generateReport
method which gets invoked after the execution of all the suites. The method further contains three parameters:- xmlSuite: it provides you with a list of multiple suites presented in the testng xml file that goes under execution.
- suites: This object represents a great deal of information about the classes, packages, test execution result, along with all the test methods. Basically, it represents detailed information around the suite after the final execution.
- outputDirectory: contains the output folder path where the report gets generated.
Below is the example of IReporterer listener at suite level.
File Name :ReporterListener.java
package TestNgListener;
import java.util.List;
import java.util.Map;
import org.testng.IReporter;
import org.testng.ISuite;
import org.testng.ISuiteResult;
import org.testng.ITestContext;
import org.testng.xml.XmlSuite;
public class ReporterListener implements IReporter {
public void generateReport(List xmlSuites, List suites, String outputDirectory) {
for (ISuite isuite: suites) {
Map & lt;
string, isuiteresult = "" & gt;
suiteResults = isuite.getResults();
String sn = isuite.getName();
for (ISuiteResult obj: suiteResults.values()) {
ITestContext tc = obj.getTestContext();
System.out.println("Passed Tests of" + sn + "=" + tc.getPassedTests().getAllResults().size());
System.out.println("Failed Tests of" + sn + "=" + tc.getFailedTests().getAllResults().size());
System.out.println("Skipped Tests of" + sn + "=" + tc.getSkippedTests().getAllResults().size());
}
}
}
} & lt;
/string,>
File Name: ReporterTest.java
package TestNgListener;
import org.testng.SkipException;
import org.testng.annotations.Listeners;
import org.testng.annotations.Test;
import junit.framework.Assert;
public class ReporterTest {
public void FirstTest() {
System.out.println("The First Test Method");
Assert.assertTrue(true);
}
public void SecondTest() {
System.out.println("The Second Test Method");
Assert.fail("Failing this test case");
}
public void ThirdTest() {
System.out.println("The Third Test Method");
throw new SkipException("Test Skipped");
}
}
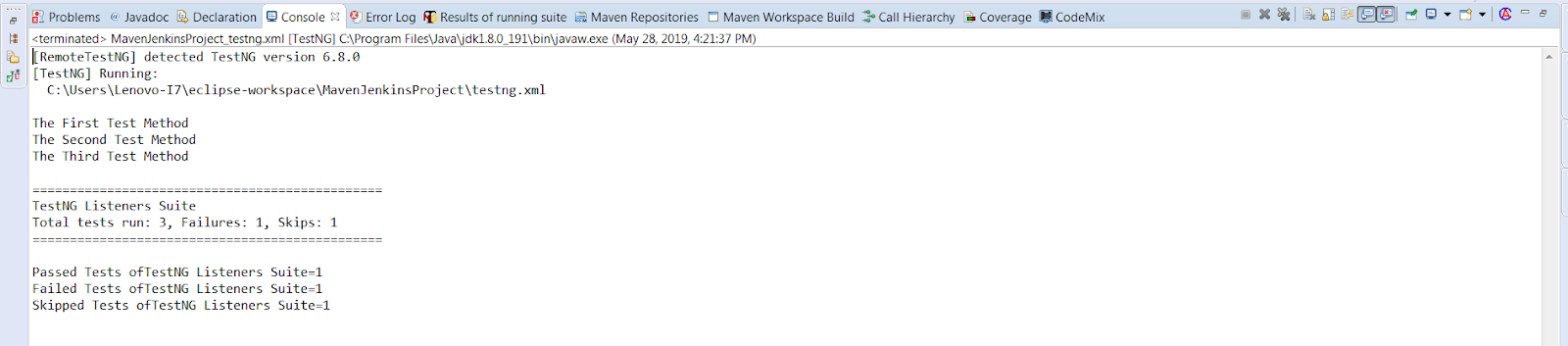
No comments:
Post a Comment